Input
POST https://gateway.appypie.com/inpainting/v1/getImage HTTP/1.1 Content-Type: application/json Cache-Control: no-cache Ocp-Apim-Subscription-Key: 8338a365bd3d4092bf6e2d9fbb54e0d1 { "prompt": "Change to a lion" }
import urllib.request, json try: url = "https://gateway.appypie.com/inpainting/v1/getImage" hdr ={ # Request headers 'Content-Type': 'application/json', 'Cache-Control': 'no-cache', 'Ocp-Apim-Subscription-Key': '8338a365bd3d4092bf6e2d9fbb54e0d1', } # Request body data = data = json.dumps(data) req = urllib.request.Request(url, headers=hdr, data = bytes(data.encode("utf-8"))) req.get_method = lambda: 'POST' response = urllib.request.urlopen(req) print(response.getcode()) print(response.read()) except Exception as e: print(e)
// Request body const body = { "prompt": "Change to a lion" }; fetch('https://gateway.appypie.com/inpainting/v1/getImage', { method: 'POST', body: JSON.stringify(body), // Request headers headers: { 'Content-Type': 'application/json', 'Cache-Control': 'no-cache', 'Ocp-Apim-Subscription-Key': '8338a365bd3d4092bf6e2d9fbb54e0d1',} }) .then(response => { console.log(response.status); console.log(response.text()); }) .catch(err => console.error(err));
curl -v -X POST "https://gateway.appypie.com/inpainting/v1/getImage" -H "Content-Type: application/json" -H "Cache-Control: no-cache" -H "Ocp-Apim-Subscription-Key: 8338a365bd3d4092bf6e2d9fbb54e0d1" --data-raw "{ \"prompt\": \"Change to a lion\" }"
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.util.HashMap; import java.util.Map; import java.io.UnsupportedEncodingException; import java.io.DataInputStream; import java.io.InputStream; import java.io.FileInputStream; public class HelloWorld { public static void main(String[] args) { try { String urlString = "https://gateway.appypie.com/inpainting/v1/getImage"; URL url = new URL(urlString); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); //Request headers connection.setRequestProperty("Content-Type", "application/json"); connection.setRequestProperty("Cache-Control", "no-cache"); connection.setRequestProperty("Ocp-Apim-Subscription-Key", "8338a365bd3d4092bf6e2d9fbb54e0d1"); connection.setRequestMethod("POST"); // Request body connection.setDoOutput(true); connection .getOutputStream() .write( "{ \"prompt\": \"Change to a lion\" }".getBytes() ); int status = connection.getResponseCode(); System.out.println(status); BufferedReader in = new BufferedReader( new InputStreamReader(connection.getInputStream()) ); String inputLine; StringBuffer content = new StringBuffer(); while ((inputLine = in.readLine()) != null) { content.append(inputLine); } in.close(); System.out.println(content); connection.disconnect(); } catch (Exception ex) { System.out.print("exception:" + ex.getMessage()); } } }
$url = "https://gateway.appypie.com/inpainting/v1/getImage"; $curl = curl_init($url); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); # Request headers $headers = array( 'Content-Type: application/json', 'Cache-Control: no-cache', 'Ocp-Apim-Subscription-Key: 8338a365bd3d4092bf6e2d9fbb54e0d1',); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); # Request body $request_body = '{ "prompt": "Change to a lion" }'; curl_setopt($curl, CURLOPT_POSTFIELDS, $request_body); $resp = curl_exec($curl); curl_close($curl); var_dump($resp);
Output
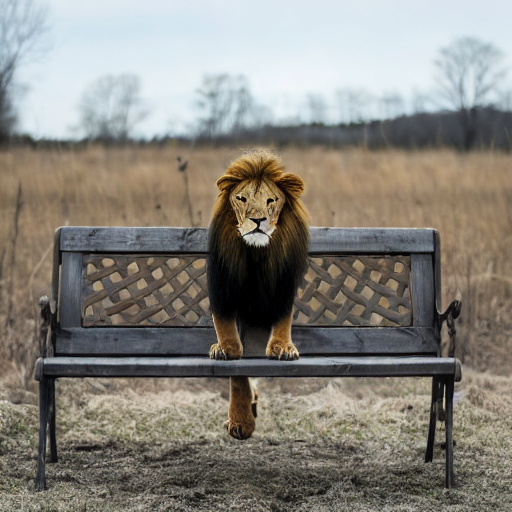
Top APIs for Generative AI Models
Unlock the full potential of your projects with our Generative AI APIs. from video generation APIs to image creation, text generation, animation, 3D models, prompt generation, image restoration, and code generation, we offer advanced APIs for all your generative AI needs.